Simple Feature Based Scheduler run with SOCSΒΆ
This example notebook show how to do a simple 1 day FBS run using SOCS. In this example we use the default FBS configuration, a separate example will be given on how to provide a custom configuration.
Before running the notebook make sure you run manage_db --save-dir $HOME/run_local/output/
on the command line to setup the SOCS database.
[1]:
import logging
import healpy as hp
from lsst.sims.ocs.database import SocsDatabase
from lsst.sims.ocs.kernel import Simulator
from lsst.sims.featureScheduler.driver import FeatureSchedulerDriver as Driver
from lsst.sims.ocs.setup import create_parser
from lsst.sims.ocs.setup import apply_file_config, read_file_config
[2]:
logging.getLogger().setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO,
format='%(asctime)s %(name)-12s %(levelname)-8s %(message)s',
datefmt='%m-%d %H:%M')
This next cell loads default command line arguments. These are needed mainly to setup the database.
[3]:
parser = create_parser()
args = parser.parse_known_args()[0]
prog_conf = read_file_config()
if prog_conf is not None:
apply_file_config(prog_conf, args)
print(args.sqlite_save_dir, args.session_id_start, args.sqlite_session_save_dir)
/home/opsim/run_local/output/ None None
Setup socs database to store simulations results, if needed.
[4]:
db = SocsDatabase(sqlite_save_path=args.sqlite_save_dir,
session_id_start=args.session_id_start,
sqlite_session_save_path=args.sqlite_session_save_dir)
[5]:
session_id = db.new_session("FBS test on notebook")
We now define a driver for the simulation. In this case, we already imported the FBS driver as Driver so we simply call it.
[6]:
driver = Driver()
By default the duration of a simulation is 10 years. Here we will run a single day.
[7]:
args.frac_duration = 0.003
We now set the SOCS simulator
[8]:
sim = Simulator(args, db, driver=driver)
[9]:
sim.initialize()
09-13 17:51 kernel.Simulator INFO Initializing simulation
09-13 17:51 kernel.Simulator INFO Simulation Session Id = 2007
09-13 17:51 configuration.ConfigurationCommunicator INFO Initializing configuration communication
09-13 17:51 kernel.Simulator INFO Finishing simulation initialization
And run the simulation
[10]:
sim.run()
09-13 17:51 kernel.Simulator INFO Starting simulation
09-13 17:51 kernel.Simulator INFO run: rx scheduler config survey_duration=3650.0
09-13 17:51 kernel.Simulator INFO run: rx driver config={'ranking': {'coadd_values': 1, 'time_balancing': 1, 'timecost_time_max': 150.0, 'timecost_time_ref': 5.0, 'timecost_cost_ref': 0.3, 'timecost_weight': 1.0, 'filtercost_weight': 1.0, 'propboost_weight': 1.0, 'lookahead_window_size': 0, 'lookahead_bonus_weight': 0.0}, 'constraints': {'night_boundary': -12.0, 'ignore_sky_brightness': 0, 'ignore_airmass': 0, 'ignore_clouds': 0, 'ignore_seeing': 0}, 'darktime': {'new_moon_phase_threshold': 20.0}, 'startup': {'type': 'HOT', 'database': ''}}
09-13 17:51 kernel.Simulator INFO run: rx site config={'obs_site': {'name': 'Cerro Pachon', 'latitude': -30.2444, 'longitude': -70.7494, 'height': 2650.0}}
09-13 17:51 kernel.Simulator INFO run: rx telescope config={'telescope': {'altitude_minpos': 20.0, 'altitude_maxpos': 86.5, 'azimuth_minpos': -270.0, 'azimuth_maxpos': 270.0, 'altitude_maxspeed': 3.5, 'altitude_accel': 3.5, 'altitude_decel': 3.5, 'azimuth_maxspeed': 7.0, 'azimuth_accel': 7.0, 'azimuth_decel': 7.0, 'settle_time': 3.0}}
09-13 17:51 kernel.Simulator INFO run: rx dome config={'dome': {'altitude_maxspeed': 1.75, 'altitude_accel': 0.875, 'altitude_decel': 0.875, 'altitude_freerange': 0.0, 'azimuth_maxspeed': 1.5, 'azimuth_accel': 0.75, 'azimuth_decel': 0.75, 'azimuth_freerange': 4.0, 'settle_time': 0.0}}
09-13 17:51 kernel.Simulator INFO run: rx rotator config={'rotator': {'minpos': -90.0, 'maxpos': 90.0, 'maxspeed': 3.5, 'accel': 1.0, 'decel': 1.0, 'filter_change_pos': 0.0, 'follow_sky': 0, 'resume_angle': 0}}
09-13 17:51 kernel.Simulator INFO run: rx camera config={'camera': {'readout_time': 2.0, 'shutter_time': 1.0, 'filter_change_time': 120.0, 'filter_max_changes_burst_num': 1, 'filter_max_changes_burst_time': 0.0, 'filter_max_changes_avg_num': 3000, 'filter_max_changes_avg_time': 31557600.0, 'filter_removable': ['y', 'z'], 'filter_mounted': ['g', 'r', 'i', 'z', 'y'], 'filter_unmounted': ['u']}}
09-13 17:51 kernel.Simulator INFO run: rx slew config={'slew': {'prereq_domalt': [], 'prereq_domaz': [], 'prereq_domazsettle': ['domaz'], 'prereq_telalt': [], 'prereq_telaz': [], 'prereq_telopticsopenloop': ['telalt', 'telaz'], 'prereq_telopticsclosedloop': ['domalt', 'domazsettle', 'telsettle', 'readout', 'telopticsopenloop', 'filter', 'telrot'], 'prereq_telsettle': ['telalt', 'telaz'], 'prereq_telrot': [], 'prereq_filter': [], 'prereq_exposures': ['telopticsclosedloop'], 'prereq_readout': []}}
09-13 17:51 kernel.Simulator INFO run: rx optics config={'optics_loop_corr': {'tel_optics_ol_slope': 0.2857142857142857, 'tel_optics_cl_alt_limit': [0.0, 9.0, 90.0], 'tel_optics_cl_delay': [0.0, 36.0]}}
09-13 17:51 kernel.Simulator INFO run: rx park config={'park': {'telescope_altitude': 86.5, 'telescope_azimuth': 0.0, 'telescope_rotator': 0.0, 'dome_altitude': 90.0, 'dome_azimuth': 0.0, 'filter_position': 'z'}}
09-13 17:51 featureSchedulerDriver INFO Loading feature based scheduler configuration from /home/opsim/repos/scheduler_config/config_run/feature_scheduler.py.
09-13 17:52 featureSchedulerDriver INFO Start up type is HOT, no state will be read from the EFD.
09-13 17:52 kernel.Simulator INFO Night 1
09-13 17:52 featureSchedulerDriver INFO start_survey t=1664580953.883245
09-13 17:52 featureSchedulerDriver INFO start_night t=1664580953.883245, night=1 timeprogress=0.00%
09-13 17:52 featureSchedulerDriver INFO start_night t=1664580953.883245, night=1 timeprogress=0.00%
/home/opsim/repos/sims_skybrightness_pre/python/lsst/sims/skybrightness_pre/SkyModelPre.py:363: UserWarning: Requested MJD between sunrise and sunset, returning closest maps
warnings.warn('Requested MJD between sunrise and sunset, returning closest maps')
/home/opsim/repos/sims_skybrightness_pre/python/lsst/sims/skybrightness_pre/SkyModelPre.py:279: UserWarning: Requested MJD between sunrise and sunset, returning closest maps
warnings.warn('Requested MJD between sunrise and sunset, returning closest maps')
/home/opsim/repos/sims_seeingModel/python/lsst/sims/seeingModel/seeingModel.py:133: RuntimeWarning: invalid value encountered in power
airmass_correction = np.power(airmass, 0.6)
/home/opsim/repos/sims_skybrightness_pre/python/lsst/sims/skybrightness_pre/SkyModelPre.py:49: RuntimeWarning: invalid value encountered in true_divide
wterm = (x - xp[left])/baseline
09-13 17:53 featureSchedulerDriver INFO end_night t=1664616576.277367, night=1 timeprogress=0.01%
09-13 17:53 featureSchedulerDriver INFO end_night next moonphase=40.78%
09-13 17:53 featureSchedulerDriver INFO end_night bright time waxing
We now have access to all the scheduler data structure to play with. In the cell bellow, we plot the TargetMapBasis function for the g
filter.
[11]:
hp.mollview(sim.driver.scheduler.survey_lists[0][1].basis_functions[2]())
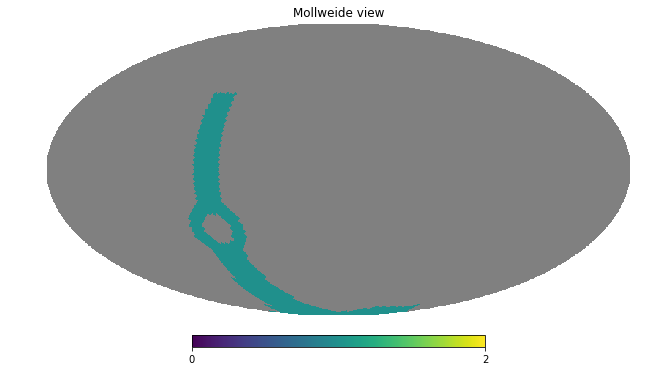
[ ]: